13.1 Introduction
In everyday life, it is common for us to make decisions based on information available to us. In order to write programs that make decisions based on data the user provides, programming languages use the “IF condition”. In Scratch, go to the Control tab to find the If-then block.
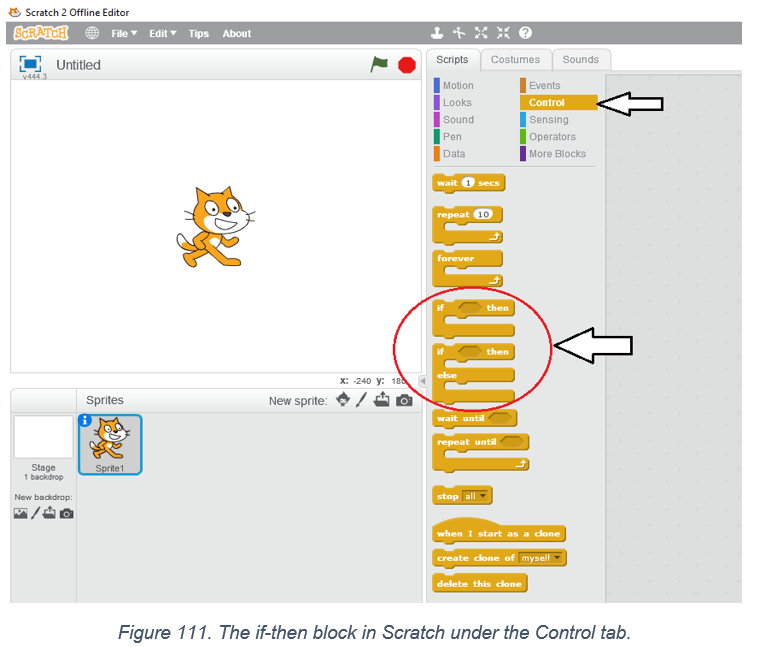
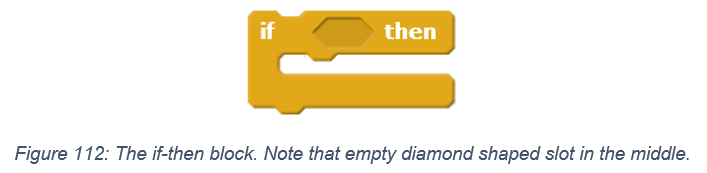
In the center of the If-then block, we can see a diamond shaped box. This empty box is filled using the different diamond shaped blocks in the Operators tab. The blocks allow us to specify the condition we want the program to check. The result of this check is always True or False, Yes or No, 0 or 1. This should sound very familiar from our previous discussion regarding binary information. In other words, the result of an If condition check is a bit The important thing to understand is that the code inside an if block is only executed when the condition it checks is True.
Let us see the If-then block in action using the “=” operator block. What if we want to tell the computer to play note 60 if two equals three. Two does not equal three and so the note will not play, but this does not stop us from stating such a condition. In a programming language, this condition is “if 2 = 3 then play note 60”. The corresponding Scratch script is in Figure.
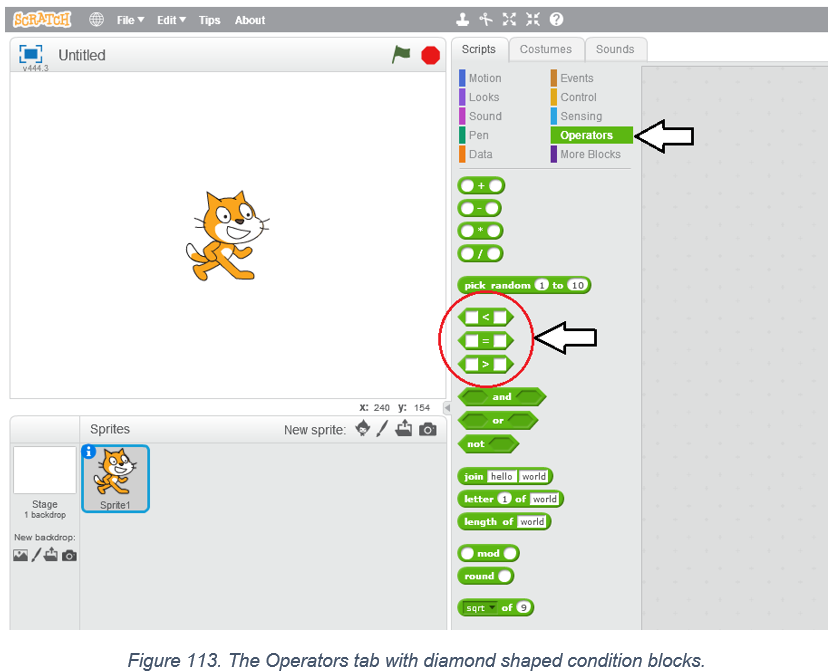
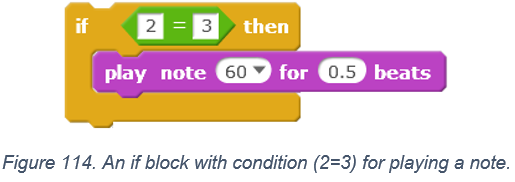
Let us try the other operator block. We can try less than. We choose to say if 3 is less than 5 then play note 60. Will it play the note? Yes, it will because our condition is True, three is indeed less than five. This all appears very simple; and it is! Some of the most complicated decisions can always be broken down into just lots of if conditions over variables within repeat blocks.
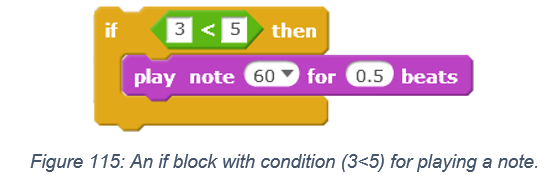
IF conditions work a lot like other blocks in Scratch in terms of how they combine. First, consider this simple example where we have a script that plays three notes, note 60, 62, and 64 in order. Try it out in Scratch.
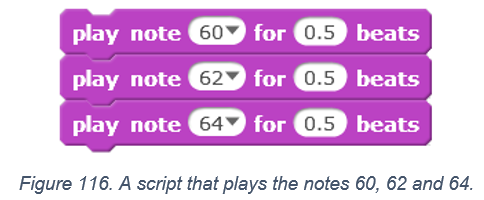
Are the following Scripts equivalent the one above?
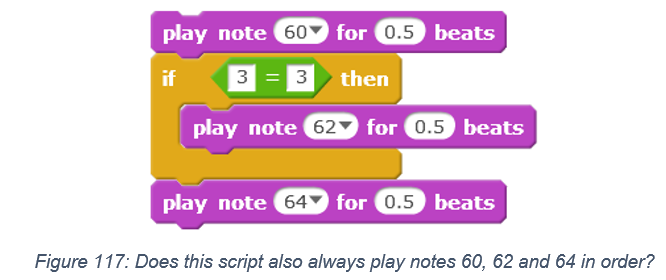
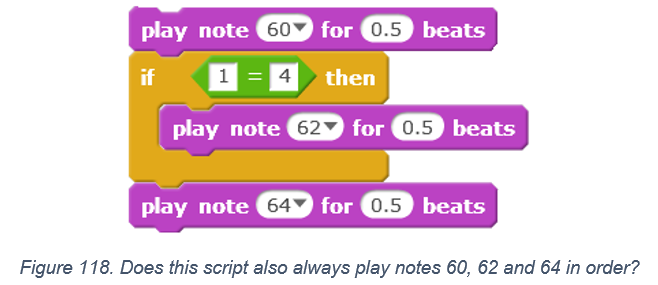
We have stated that the result of an if condition is binary, yet so far we have only seen how to tell Scratch to do something when the result of the condition is True. How do we ask Scratch to do something only when if condition if False? This requires the If-then-Else condition block as shown in Figure 119. It looks really similar to the If-then block, except that now we can add code inside the “else” case, which is exactly what gets executed when the condition is False.
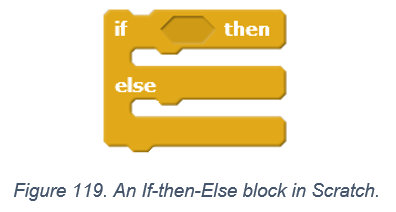
Consider the script in Figure 120. We can read it as, “if 3 is less than 5 then play note 60, and if it not, i.e., (else) play drum 1”. Play it in Scratch.
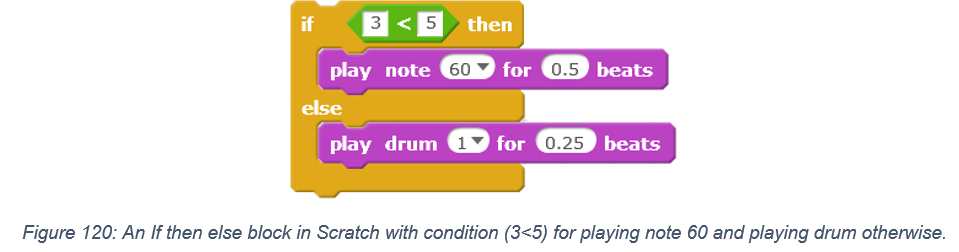
Now, check what the outcome is when we change the condition to . We understand the If-then-Else block as follows. If condition is True then execute this code else if condition is False execute the following code.
Exercises
- Will the note 60 play when the following script runs?
- Will the note 60 play when the following script runs?
- What will play when the following script is executed?
- What will play when the following script is executed?
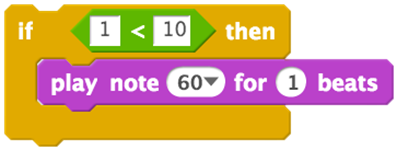
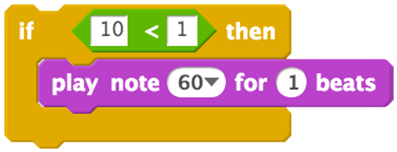
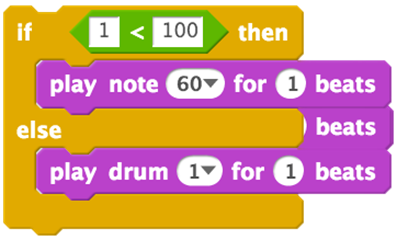
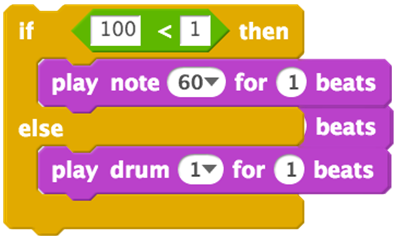
13.2 Multiple IF blocks
Just like we could combine multiple repeat blocks, let us look at the result of combining multiple If blocks.
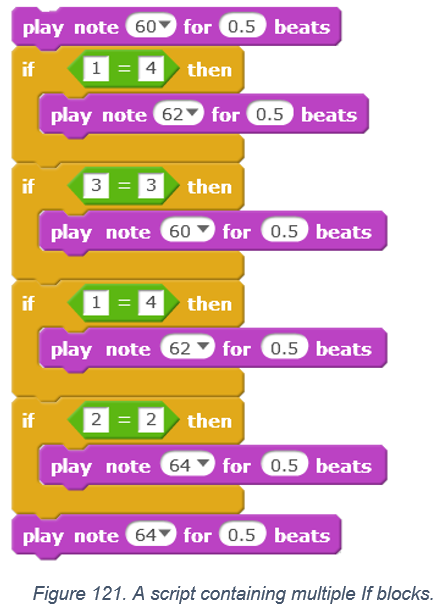
What are the sequence of notes played when the above script executes? The answer is 60, 60, 64, 64. Trace through the code to verify the answer.
Another way we can combine the conditions is to put one “if” block inside another “if” block, i.e., nesting them. This is similar to how we have previous send nested repeats. This allows us to check multiple conditions before executing a specific piece of code. Note that even if one condition is True, a following “if” condition inside the previous “if” condition may not be True.
What does the Sprite do when we run the script in Figure 122? The sprite first moves 50 blocks. The first if condition is satisfied as well as the second condition, which results in play drum one being executed.
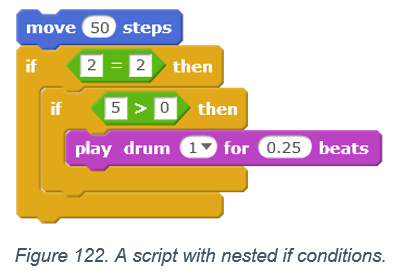
The following observation helps in tracing nested if conditions. If an outer “if” condition is not True then any block within this if block is ignored. Try out the example below.
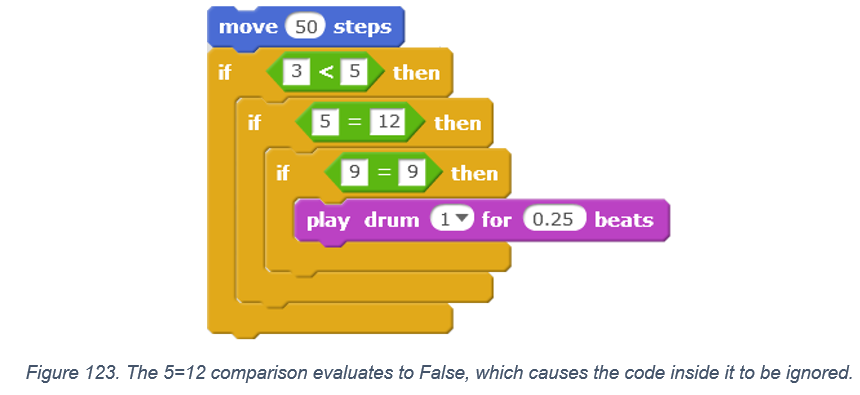
When “if” conditions are used sequentially, they are not dependent on each other. If they are nested then the inner “if” blocks depend on the conditions of the outer ones.
Exercises
- What notes are played (if any) when you double click the script below? Check all that apply.
- 60
- 61
- Nothing will play
- Can you predict how many steps will the Sprite move if we double click the script below?
- 20 and then 100 steps
- 50 then 20 then 100 then 30
- 100 steps only
- Will the drum play when we run the script given below?
- Yes
- No
13.3 Using If conditions with Variables
If conditions provide a lot of flexibility when we use them with variables. Your programs can perform interesting things when you combine them. Rather than fixing a value in the if condition, we can now have the decision depend on a value that may be calculated or is provided by the user as input. Let us look at an example.
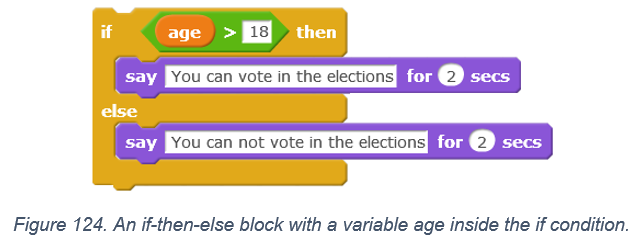
The “if” condition in Figure 124 depends on the variable age. The scripts makes the sprite say a different message depending on whether the value in the variable age passes the criteria. If it is above 18, then the user is allowed to vote in elections and otherwise the user cannot vote in elections. Note that the outcome of this script can be different each time we run it, based on the value of the variable age. Test running the script with different values of the age variable.
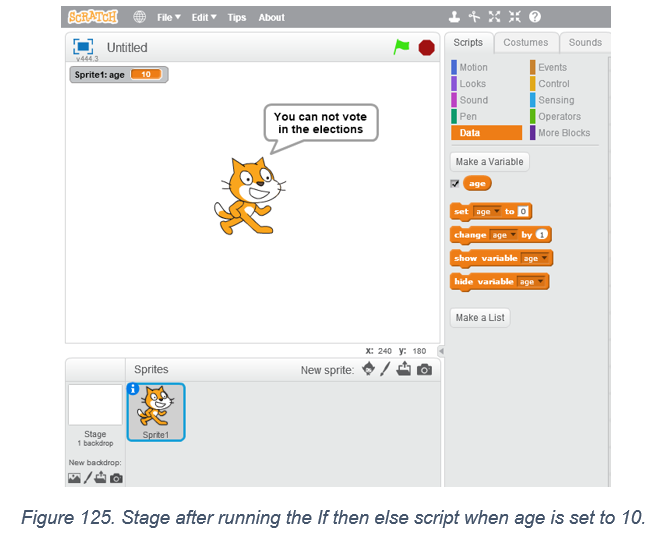
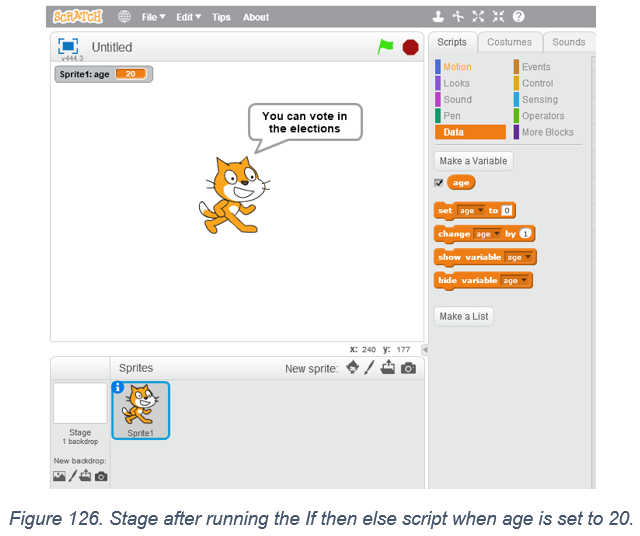
Let us look at another example. Now we put the set block right on top of the If-then-else block. This allows you to quickly test the script for different values of the variable x. Try it out in Scratch!
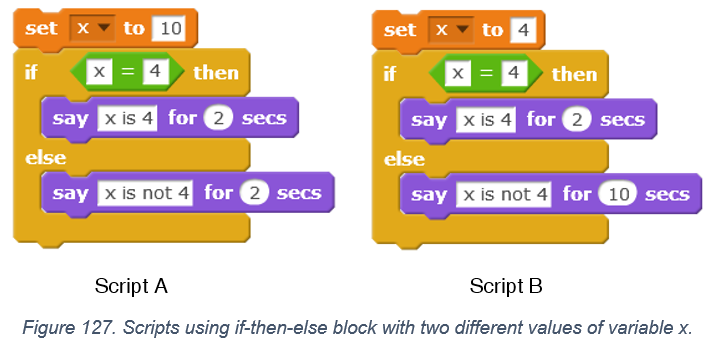
The script in Figures 128, 129 and 130 show how we can use the “if” condition to change the value of one variable based on the value of another variable. We start by fixing the value of variables y and x. Next, our conditional action is to change the value of y by one if x equals four. If x does not equal four then the value of y is changed by two. What is the value of y after these scripts execute?
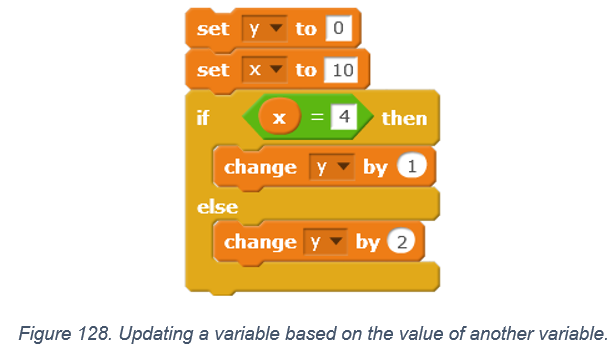
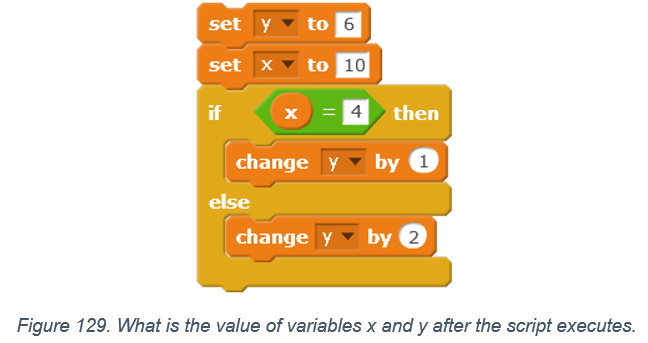
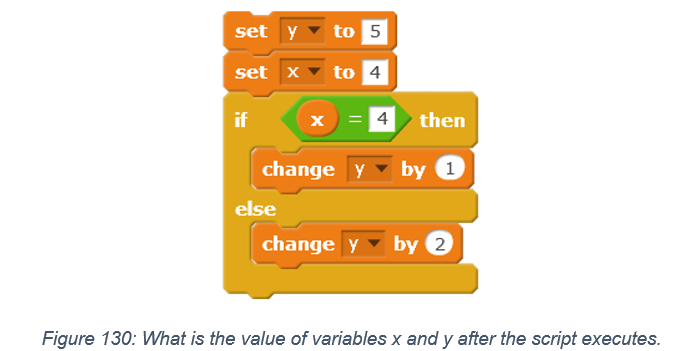
Exercises
What is the value of x after running the scripts below?
What is the value of the variables x and age after running the script below?
There is basket, which needs to be filled with 15 apples. Consider the following script with two variables: apple and basket.
What is the output of the script when we run it for the first time?
- basket = 1, apple = 1
- basket = 0, apple = 1
- basket = 0, apple = 0
What is the value of “my length” and “x” after the script runs?
What is the value of “cost of soda” and “cost of pizza” after the script runs?
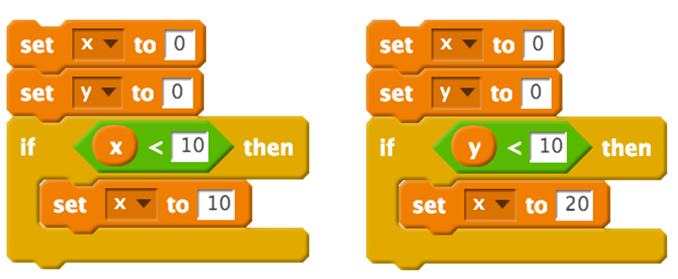
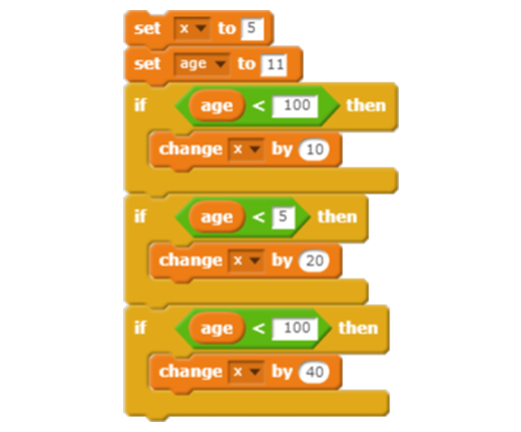
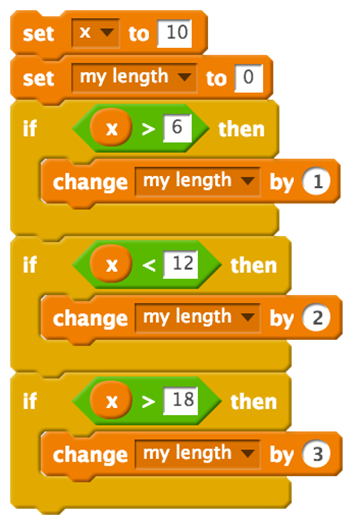
a. my length = 0 b. my length = 3 c. my length = 6 d. my length = 10
a. x = 0 b. x = 3 c. x = 6 d. x = 10
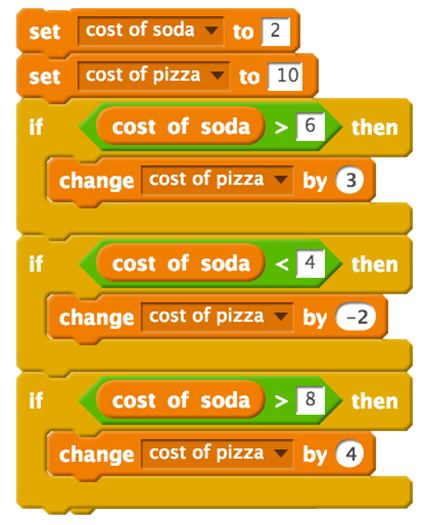
a. cost of soda = 2 b. cost of soda = 3 c. cost of soda = 5 d. cost of soda = 6
a. cost of pizza = 8 b. cost of pizza = 9 c. cost of pizza = 13 d. cost of pizza = 14